常見的 JavaScript 函數
• console.log() - 將 message 輸出到 Web 控制台。 message 可以是單個 string,也可以是任何一個或多個 JavaScript Object。
• window.alert() - 指示瀏覽器顯示帶有可選消息的對話框,並等待用戶關閉對話框。
• window.prompt() - 指示瀏覽器顯示帶有可選消息的對話框,提示用戶輸入一些文字,並等待用戶提交文字或取消對話框。
Lexical Structure
程式語言的 Lexical Structure 是一組基本規則,用於指定如何用該語言編寫程式。它是一種語言的最低級語法,例如:它指定變量名稱的外觀、註釋的分隔符以及如何將一個程式語句與下一個程式語句分開。以下為 JavaScript 中的幾個 Lexical Structure:
1. Case Sensitive - JavaScript 中的大小寫是有差別的。
2. 空白鍵、換行鍵等等在 JavaScrip t當中會全部被忽略。通常來說,放到伺服器上的 JavaScript 程式碼都會被做 minification (刪除空白鍵、換行鍵)。Minification 可少量的減少 JS檔案大小。
3. JavaScript 的當行註解是 //,多行則是包裹在 /**/ 內部。
4. 在 JavaScript 內部的變數名稱需要由文字、underscore()、dollar sign($) 當作開頭 (不能用數字開頭)。
5. JavaScript 內部有關鍵字 (reserved words, keywords),例如:null、of、 if、then、in、finally、 for、while、break、 continue、switch、try、let、 const、var 等等,不能當作變數名稱。
6. JavaScript 使用 Unicode 字元集合,所以 String 內部可由任何 Unicode 文字組成。
7. Semicolons(;) 可用來分隔程式語句。 Semicolons 的使用是 optional。
變數 (variable) 與賦值 (assignment)
- 變數 (variable) 和賦值 (assignment) 是任何程式語言中的一些基本概念。變數是一個可以存儲值的容器。由於變數內部的值可以不斷改變,它被稱之為「變數」。
- JavaScript 當中的等號與數學中使用的等號概念不同!在JavaScript 中,等號是 “賦值” (assignment),意思是要把等號右邊的數據放到等號左邊,因此,我們可以這樣做:
x = 5, x = x + 1
經過執行之後,x 的值會變成 6。
*. 語法糖 (syntax sugar) 支援將 x=x+1 更改為 x+=1。 這在 JavaScript 中極為常見。
- 當我們想要在 JavaScript 創造一個變數,我們需要宣告變數(declare variable)。宣告變數之前,我們要先確認,此變數之後是否有可能被修改。例如,數學中的 π = 3.14159,是不會變動的。銀行存款卻會不斷變動。基本規則:
1. 若變數的值會變動,則用 let 來宣告變數。
2. 若變數的值不會變動,則用 const 來宣告變數。
3. 請勿使用 var 宣告變數。
幾個需要特別注意的規則:
1. 用 const 來宣告的變數,一定需要馬上賦予初始值 (initializer)。let 則不需要。若用 let 宣告了變數,但還沒有賦值,則變數的值是 undefined。
2. 用 const、let 宣告過的變數,都不能重複宣告。(redeclaration is not allowed)。
3. const 不能做重複賦值 (reassignment is not allowed)

*. JavaScript 引擎中有一個稱為 garbage collector 的後台程式。它監視所有對象並刪除那些變得無法訪問的 object。
Data Type - primitive data type
1. Number - 整數或帶小數點的。
2. BigInt - 任意長度的整數。
3. String - 字符串。
4. Boolean - true 或 false 兩種值。
Unary operator - ! ,可以將 Boolean 值反轉。
Unary operator - typeof ,可以用來確認資料型態。
5. null - 用來代表故意不存在的值。
6. undefined
- 已經宣告變數,卻沒有賦予 initializer 時,就會出現 undefined。
- undefined 也是 JavaScript 中的 function 的預設 return value。
Number Method
1. toString()
return 一個數字的 String。
2. toFixed(n)
return 被轉換後的數字,到小數點後第 n 位數 (注意:轉換後的結果是 string)。
(Note:二進制不能精確的表示所有小數,例如:0.1 + 0.2 不會等於 0.3)
String Attributes and Methods
string.length
- return String 的長度
string[n]
- return index 第 n 項的字。(index 從 0 開始計算)
string.slice(indexStart[, indexEnd])
- 提取字符串的一部分將其作為新的 String 返回。indexStart 是 inclusive (包含),indexEnd 是 optional, exclusive (不包含)。
indexOf(substring)
- return sbusting 的 index 位置,若找不到 substring,則 return -1。
toUpperCase()
- return 轉換為大寫的 String。
toLowerCase()
- return 轉換為小寫的 String。
startsWith(s)
- 確定 String 是否以指定字串 s 開頭,return true 或 false。
endsWith(s)
- 確定 String 是否以指定字串 s 結尾,return true 或 false。
include(str)
- 如果 String 內部包含 str,return true
charCodeAt(n)
- return 一個介於 0 和 65535 之間的整數,表示給定索引處 n 的 UTF-16 code unit。 reference:ASCI
split(pattern)
- 接受一個 pattern 並通過搜索將一個字符串切成一個有序的 array,然後 return 該 array。
範例:
let sentence = "Today is a good day";
let result = sentence.split(" "); // 從空白處切一刀
console.log(result);
結果為:["Today", "is", "a", "good", "day"]
JavaScript Operators
A. assignment operator:
let x = 5;
B. comparison operator:
comparison operators 的運算元 (operands) 是兩個任意資料型態,且運算結果為 Boolean 值。
1. == returns true if the operands are equal.
2. != returns true if the operands are not equal.
3. === returns true if the operands are equal and of the same data type.
4. !== returns true if the operands are of the same data type but not equal, or are of different type.
5. > returns true if the left operand is greater than the right operand.
6. >= returns true if the left operand is greater than or equal to the right operand.
7. < returns true if the left operand is less than the right operand.
8. <= returns true if the left operand is less than or equal to the right operand.
範例:
console.log(3 == "3");
結果為:true,檢查 operands 的值。
console.log(3 === "3");
結果為:false,檢查 operands 的值,也檢查 data type。
C. logical operator
logical operators 的運算元 (operands) 也是兩個任意資料型態,且運算結果為 Boolean 值。
&& => and
|| => or
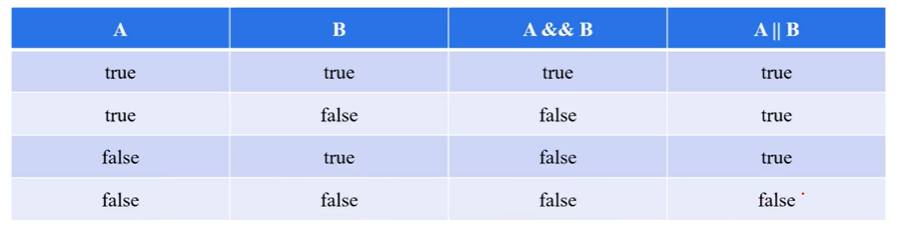
D. typeof operator(unary)
E. negation operator(unary):!
F. increment operator
例如:x++, x--
G. bitwise operator
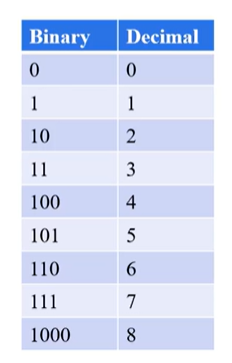
1. a & b :在兩個 operand 的對應位都是 1 的位置返回一個 1。
範例:
let a = 10;
let b = 9;
console.log(a & b);
結果為:8。
二進位運算:
10 => 1010
9 => 1001
運算後得到:1000 => 8
2. a | b :在兩個 operand 的對應位都是0 的位置返回一個 0。
範例:
let a = 10;
let b = 9;
console.log(a | b);
結果為:11。
二進位運算:
10 => 1010
9 => 1001
運算後得到:1011 => 11
3. a ^ b :(XOR 運算) 在兩個 operand 的對應位相同的每個位置返回一個 0。
範例:
let a = 10;
let b = 9;
console.log(a ^ b);
結果為:3。
二進位運算:
10 => 1010
9 => 1001
運算後得到:0011 => 3
H. arithmetic operator
例如:+, -, *, /, +=, -=, /=, *=, **, %
if statement
語法:
a. if (condition) {statement1;}
b. if (condition) {
statement1;
} else {
statement2;
}
c. if (condition) {
statement1;
} else if {
statement2;
} ...
else {
statementN;
}
範例:
let age = prompt("請輸入你的年齡:(阿拉伯數字)");
age = Number(age); // convert string to number
if (age >= 0 && age <= 12) {
alert("您的兒童票一張100元");
} else if (age > 12 && age <= 65) {
alert("您的成人票一張250元");
} else if (age > 65 && age <= 125) {
alert("您的敬老票一張150元");
}
Truthy and Falsy Values
Falsy Values:
除了上述之外,都是 truthy values,包含 [] empty array, {} empty object 等等。
補充:
&&
a. If the left hand side is true, then it evaluates as the right hand side.
b. if the left hand side is not true, then it evaluates as the left hand side.
範例 a:
console.log(-3 && -10);
結果為:-10。
範例 b:
console.log(0 && -100);
結果為:0。
||
a. If the left hand can be converted to true, return left hand side.
b. else, return right hand side.
範例 a-1:
console.log(6 || 100);
結果為:6。
範例 a-2:
let data = "Wilson"
console.log(data || "資料讀取失敗");
結果為:Wilson。
範例 b-1:
console.log(NaN || 100);
結果為:100。
範例 b-2:
let data = ""
console.log(data || "資料讀取失敗");
結果為:資料讀取失敗。
JavaScript Function 函式
除了 JavaScript 內建的 function 之外,我們可以定義或客製化自己的 function。Function 由一連串的程式碼組成,用來完成任務或是計算值。
宣告 (declare )一個 Function 的語法為:

return 關鍵字
JavaScript Function 中,若沒有 return 語句的話, function 將返回 undefined (這是 JavaScript 的 function 默認的 return value)。要返回默認值以外的值,函數必須具有指定要返回的值的 return 語句。
return 語句結束函式執行並指定要返回給函數調用者的值。任何放在 return 語句底下的程式碼都不會被執行。
Function執行、調用的英文是 function call、function execution 或是 function invocation
*. 每個 JavaScript 函數實際上都是一個物件。(代表每個 function 有 instance properties 以及instance methods)
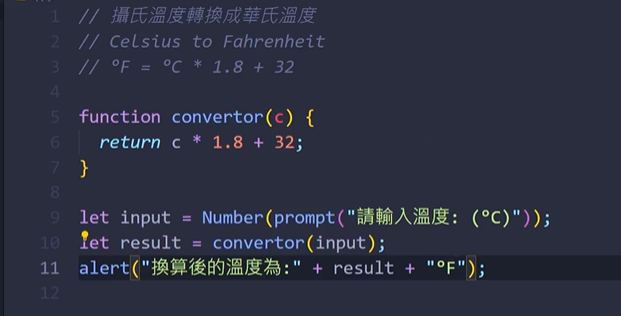
Array (陣列)
- array 並不是 primitive data type
- array 複製是依據 reference 複製,(同一個 RAM 位置) - reference data type
範例 a:primitive data type
let x =10;
let y = 10;
console.log(x == y);
結果為:true。
範例 b:reference data type
let arr1 = [1, 2, 3];
let arr2 = [1, 2, 3];
console.log(arr1 == arr2);
結果為:false,兩個 array分別處於不同的 RAM 位置。
Array instance property - length
arr.length
let arr = ["a", "b", "c"];
console.log(arr.length);
結果為:3
Array instance Methods:(常見的4種)
push(element) - 將一個或多個元素添加到陣列的末尾,並 return 陣列的新長度。
let arr = ["a", "b", "c"];
// arr.push("d");
// arr.push("d", "e");
pop() - 從陣列中刪除最後一個元素並返回該元素。
let arr = ["a", "b", "c"];
let mypop = arr.pop();
console.log(mypop);
結果為:c
console.log(arr);
結果為: ["a", "b"]
shift() - 從陣列中刪除第一個元素並返回刪除的元素。
let arr = ["a", "b", "c"];
let myshift = arr.shift();
console.log(myshift);
結果為:a
console.log(arr);
結果為: ["b", "c"]
unshift(element) - 將一個或多個元素添加到陣列的開頭,並 return 陣列的新長度。
let arr = ["a", "b", "c"];
// arr.unshift("aa");
// arr.unshift("aa", "bb");
array of arrays - 當 array 內部元素還有 array 時
let myarr = [
["name", "address", "age"],
["Mike", "Taiwan", 35],
["Grace", "USA", "26"],
];
console.log(myarr[1][1]); // Taiwan
console.log(Array.isArray(myarr)); // True ---- check arr 是否為 array
array 串連的方法
concat()
let num1 = [1, 2, 3];
let num2 = [4, 5, 6];
let result = num1.concat(num2);
console.log(result); // [1, 2, 3, 4, 5, 6]
Object
- 每個 JavaScript 物件都有 properties 以及 method,屬於物件的 function 被稱為 method。
- 物件的屬性與相對應的值是一種 key-value pair。獲取物件屬性的方式可以透過 dot notation 或是 []。
- 在 method 中的 this 關鍵字指的是調用該方法的物件。若某個 function 沒有調用該 function 的物件,則 this 關鍵字則是指向 window 物件。
- 在 JavaScript 當中的 function、array 其實都是物件(Object)。Array 以及 Function 都是 special type of objects。
範例:
let Wilson = {
//properties (key-value pair), methods
first_name: "Wilson",
last_name: "Ren",
age: 26,
is_married: true,
spouse: "Grace",
sayHi() {
console.log(this.first_name + " says hi");
},
walk() {
console.log(this.first_name + " is walking...");
},
speak() {
console.log(this.first_name + " says " + words);
},
};
Wilson.sayHi();
Wilson.walk();
console.log(Wilson.first_name);
console.log(Wilson["first_name"]);
Loop (迴圈)
- 若把 return 關鍵字放到 loop 內部,則循環會馬上停止。
For Loop (常使用在: 已知會循環幾次時)
語法:
for (initialization; condition; final expression) {
statement
}
Initialization 是在循環開始之前的計數器變量聲明 (declare a variable)。
Condition 是每次循環迭代之前要評估的表達式。如果此表達式的計算結果為真則執行 statement。如果 condition 的計算結果為假,則執行退出循環並轉到 for loop 之後的第一條語句。
Final expression 是在每次循環迭代結束時要執行的程式碼。一般用於更新或遞增計數器變量。
範例 1:
for (let i = 1; i <= 10; i++) {
//console.log(i);
if (i == 3) {
break; // 終止迴圈
}
console.log(i);
}
範例 2:
for (let j = 1; j <= 10; j++) {
if (j == 3) {
continue; // 跳過 continue 語句之後的循環內代碼,強制執行循環的下一個迭代。
}
console.log(j);
}
範例 3: for loop 跑 array
let arr = ["Mike", "Grace", "Jason", "Jared"];
for (let i = 0; i < arr.length; i++) {
console.log(arr[i] + " is my friend.");
}
While Loop (常使用在: 不知會循環幾次時)
while 語句創建一個循環,只要測試條件評估為真,該循環就會執行指定的語句。
語法:
while (condition) {
statement
}
範例 :
let i = 1;
while (i < 11) {
console.log(i);
i++;
}
Do while Loop
- Do while Loop 特點在於先執行語句後再評估條件。
- Do while Loop 創建一個循環,該循環執行指定的 statement,直到測試條件評估為假。
語法:
do {
statement
} while (condition);
範例 :
let i = 0;
do {
console.log(i);
i++;
} while (i < 10);
Nested Loop 巢狀迴圈
- 在 loop 內部還有另一個 loop。
- 可以使用 return 關鍵字來終止 nested loop。(需將 nested loop 包裹在 function 裡面再使用 return 關鍵字來終止 nested loop)
範例 :
let counter = 0;
for (let i = 0; i < 100; i++) {
for (let j = 0; j < 500; j++) {
counter++;
}
}
console.log(counter);
// 100 * 500 = 50000
Math Object
- Math 是一個 JavaScript 內建物件,它具有數學常數和函數的屬性和方法。
常用的 static properties:
Math.PI; // 3.141592653589793
Math.E; // 2.718281828459045
常用的 static method:
Math.pow(3, 2); // 3 的 2 次方 = 8
Math.random(); // 數字介於 0 - 1 之間 (包含 0, 但不包含 1)
Math.sqrt(2); // 開根號
Math.abs(-3); // 絕對值
Math.floor(2.7); // 無條件消去
Math.ceil(1.1); // 無條件進位